[Work Summary] Python技巧知识
Python 技巧 知识 work Summary
2023-09-11 14:22:53 时间
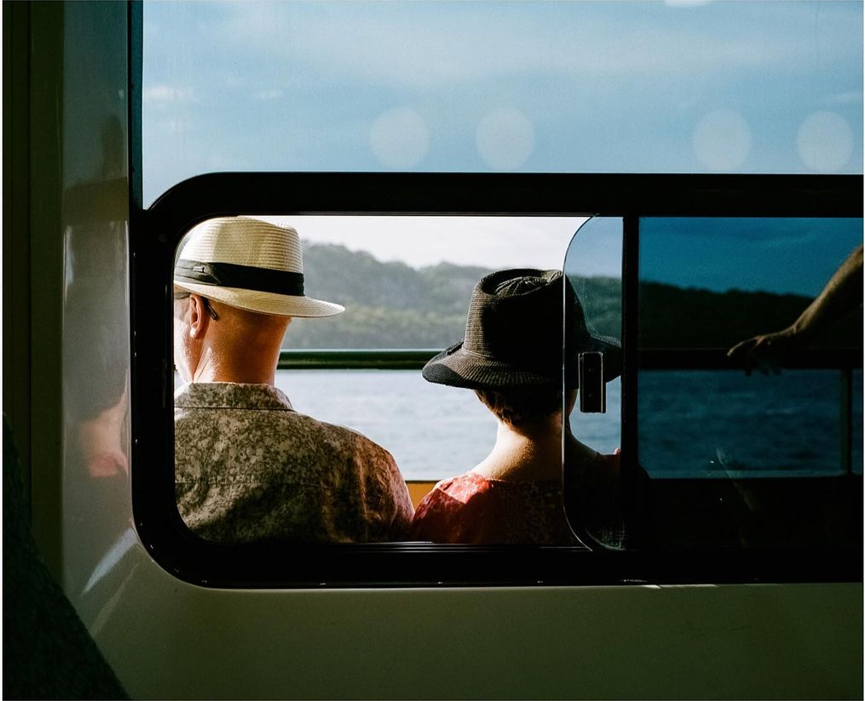
1.列表转换成字符串
aList = ["Hello", "World", "!"]
# 'Hello World !'
" ".join(aList)
2.两个列表转换成字典
keys = ['a','b','c']
values = [1,2,3]
# {'a': 1, 'b': 2, 'c': 3}
dict_nums = dict(zip(keys,values))
2.字典合并
dict1 = {'a':1,'b':2}
dict2 = {'c':3, 'd':4}
# {'a': 1, 'b': 2, 'c': 3, 'd': 4}
concat_dict = {**dict1, **dict2}
3.复制列表
nums = [1,2,3]
# [1, 2, 3]
copy_nums = nums[::]
4.反转列表
nums = [1,2,3]
# [3, 2, 1]
reverse_nums = nums[::-1]
5.列表拆包
target = [9.0, -52.0, -52.0]
a_target, b_target, c_target = target
print(a_target) # 9.0
print(b_target) # -52.0
print(c_target) # -52.0
6.交换变量
a = 1
b = 2
a, b = b, a
7.字典通过值value反查键key
dict_nums = {'a': 1, 'b': 2, 'c': 3}
# 'a'
list(dict_nums.keys())[list(dict_nums.values()).index(1)]
# 'b'
list(dict_nums.keys())[list(dict_nums.values()).index(2)]
# 'c'
list(dict_nums.keys())[list(dict_nums.values()).index(3)]
将上述公式进行逐一拆解
dict_nums = {'a': 1, 'b': 2, 'c': 3}
# 拆解list(dict_nums.keys())[list(dict_nums.values()).index(1)]
# dict_keys(['a', 'b', 'c'])
dict_nums.keys()
# ['a', 'b', 'c']
list(dict_nums.keys())
# dict_values([1, 2, 3])
dict_nums.values()
# [1, 2, 3]
list(dict_nums.values())
# 0
list(dict_nums.values()).index(1)
# 等价于['a', 'b', 'c'][0] => 'a'
list(dict_nums.keys())[list(dict_nums.values()).index(1)]
8. 删除列表中的重复元素
nums = [4,3,7,4,3,7,1,9,9,6]
unique_nums = list(set(nums))
# [1, 3, 4, 6, 7, 9]
print(unique_nums)
9.查询列表中重复最多的元素
nums = [1, 2, 3, 1, 2, 1]
most_repeated_value = max(nums, key=nums.count)
# 1
print(most_repeated_value)
10. 统计字频
# 判断字符串每个元素出现的次数,可以用collections模块中的Counter方法来实现
from collections import Counter
result = Counter('banana')
# Counter({'a': 3, 'n': 2, 'b': 1})
print(result)
11.计算字符串中某字符出现次数
# 检查字符'a'在sentence中出现的次数
sentence = 'Canada is located in the northern part of North America'
# 方法1
import re
counter = len(re.findall("a", sentence))
print(counter) # 6
# 方法2
counter = sentence.count('a')
print(counter) # 6
# 方法3
from collections import Counter
counter = Counter(sentence)
print(counter['a']) # 6
12. 检查字符串是否为空
username1 = ""
# 方法1:使用字符串长度判断
if len(username1) == 0:
print("用户名不能为空")
# 方法2:使用==判断是否为空
if username1 == '':
print("用户名不能为空")
# 方法3:直接判断是否为空
if username1:
print("Welcome!!")
else:
print("用户名不能为空")
username2 = " "
# 使用.isspace()判断是否字符串全部是空格
if username2.isspace() == True:
print("用户名不能为空")
13.字符串类型转换(将str变量类型转换为float、int或boolean)
# String to Float
float_string = "211.1996"
print(type(float_string)) # <class 'str'>
string_to_float = float(float_string)
print(type(string_to_float)) # <class 'float'>
# String to Integer
int_string = "437"
print(type(int_string)) # <class 'str'>
string_to_int = int(int_string)
print(type(string_to_int)) # <class 'int'>
# String to Boolean
bool_string = "True"
print(type(bool_string)) # <class 'str'>
string_to_bool = bool(bool_string)
print(type(string_to_bool)) # <class 'bool'>
14.将字符串拆分为Python中的字符列表
word = 'China'
l = list(word)
print(l) # ['C', 'h', 'i', 'n', 'a']
15.查找字符串中某字符的所有位置
test = 'canada#japan#china'
target_character = '#'
# [6, 12]
print([pos for pos, char in enumerate(test) if char == target_character])
16.检查字符串是否以"xxxx"开头
exp_str = "Hello World!!"
# 方法1
import re
if re.match(r'^Hello', exp_str):
print(True)
else:
print(False)
# 方法2
result = exp_str.startswith("Hello")
print(result) # True
17. 检查字符串是否以"xxxx"结尾
txt = "China is a great country"
result = txt.endswith("country")
# True
print(result)
18.替换字符串中的多个子字符
sentence = "The lazy brown fox jumps over the lazy dog"
for words in (("brown", "red"), ("lazy", "quick")):
sentence = sentence.replace(*words)
# The quick red fox jumps over the quick dog
print(sentence)
19.字符串大小写转换
str1 = "hello world!!"
# 把所有字符中的小写字母转换成大写字母
print(str1.upper()) # HELLO WORLD!!
# 把所有字符中的大写字母转换成小写字母
print(str1.lower()) # hello world!!
# 把第一个字母转化为大写字母,其余小写
print(str1.capitalize()) # Hello world!!
# 把每个单词的第一个字母转化为大写,其余小写
print(str1.title()) # Hello World!!
str2 = "blog.csdn.net"
# BLOG.CSDN.NET
print(str2.upper())
# blog.csdn.net
print(str2.lower())
# Blog.csdn.net
print(str2.capitalize())
# Blog.Csdn.Net
print(str2.title())
20.去除字符串中的space字符
str1 = " Andy437 "
# 去除首尾空格
print(str1.strip()) #Andy437
# 去除左边空格
print(str1.lstrip()) #Andy437
# 去除右边空格
print(str1.rstrip()) # Andy437
21.进制转换
# 十进制转换为二进制
# '0b1100'
bin(12)
# 十进制转换为八进制
# '0o14'
oct(12)
# 十进制转换为十六进制
# '0xc'
hex(12)
22.整数与ASCII互转
# 十进制整数对应的ASCII字符
# 'A'
chr(65)
# 某个ASCII字符对应的十进制数
# 65
ord('A')
23.元素/对象检测真假
所有元素都为真,返回True,否则返回False
# False
all[0,1,2,3]
# True
all[1,2,3]
至少有一个元素为真返回True,否则返回False
# False
any([0,0,0,[]])
# True
any[0,0,1]
测试一个对象是True还是False
# False
bool([])
# True
bool([0,0,0])
# True
bool([1,1,0])
24. 多次使用打印语句
a, b, c = 11, 12, 13
'''
11
12
13
'''
print(a, b, c, sep='\n')
25.循环打印相同的变量
repeat = 3
words = 'ABC'
# ABCABCABC
print(words * repeat)
26.检索列表中是否存在指定元素
char_list = ["A","B","A","D","C","B","E"]
search_char = "D"
# True
search_char in char_list
27.巧用zip()函数
list1 = [1,3,5,7,9]
list2 = [2,4,6,8,10]
'''
value1 = 1 value2 = 2
value1 = 3 value2 = 4
value1 = 5 value2 = 6
value1 = 7 value2 = 8
value1 = 9 value2 = 10
'''
for i, j in zip(list1, list2):
print("value1 =", i, "value2 =", j, sep=" ")
28.生成两个列表的所有组合
给定两个列表(a的长度为n,b 的长度为 m),生成 (n*m)个组合
from itertools import product
list1 = ["A", "B", "C"]
list2 = [1, 2]
combinations = list(product(list1, list2))
# [('A', 1), ('A', 2), ('B', 1), ('B', 2), ('C', 1), ('C', 2)]
print(combinations)
29.更改列表中所有元素的数据类型
给定一个表示整数的字符串列表,目标修改数据类型将其转换为整数列表
input_list = ["7", "2", "13", "15", "0"]
# map()函数接收的第一个参数是function(int),第二个参数是可迭代对象(input_list)
output_list = list(map(int, input_list))
# [7, 2, 13, 15, 0]
print(output_list)
另一种实现方式
input_list = ["7", "2", "13", "15", "0"]
output_list = []
for idx, i in enumerate(input_list):
output_list.append(int(input_list[idx]))
# [7, 2, 13, 15, 0]
print(output_list)
30.将多个子列表展开
nums = [[1,2,3],[4,5],[6]]
# [1, 2, 3, 4, 5, 6]
print([i for z in nums for i in z])
另一种实现方式
nums = [[1,2,3],[4,5],[6]]
li = []
for i in nums:
for j in i:
li.append(j)
# [1, 2, 3, 4, 5, 6]
print(li)
31.字符串拼接 f-string
name = 'Andy'
age = 18
address = '广州'
info = f'我的名字是{name},年龄是{age},来自{address}'
# 我的名字是Andy,年龄是18,来自广州
print(info)
相关文章
- python学习总结
- python 保存图片_用Python从视频中提取每一帧的图片
- Python unicode编码转中文
- Python 配置文件之ConfigParser模块(实例、封装)
- python能做什么软件?Python到底能干嘛,一文看懂
- Python零基础入门,2分钟带你了解python
- 普通人能学python吗,有何用处?
- 35岁了转行python可以吗?什么样的人合适学习Python?
- 学习python的好办法,3个实用的小技巧
- python基础——模块
- 08 python - 数据类型转换
- python os模块技巧
- Python 教程之 Python中的高级交互式仪表板,连接不同的 API 以创建用于分析的高级交互式仪表板
- 99%的Python用户都不知道的f-string隐秘技巧
- Jenkins持续集成实战之Jenkins构建Python项目提示:'python' 不是内部或外部命令,也不是可运行的程序。
- 【Python分布式服务框架】python实现gRPC服务
- python集合
- 华为OD机试 - 静态扫描最优成本(Python)| 真题+思路+考点+代码+岗位
- 华为OD机试 - 箱子之形摆放(Python)| 真题+思路+考点+代码+岗位
- python:校验邮箱格式
- 【爬虫系列】【Python】python with as的用法
- 【机器学习】神经网络BP理论与python实例系列
- Python-log() 函数