C++ 中的friend类和friend函数
0.概述
友元类可以访问其他类中声明为友元的私有成员和受保护成员。允许特定类访问其他类的私有和受保护成员时很有用。友元的主要作用也在于此。
友元是一种定义在类外部的普通函数或类,但它需要在类体内进行说明,为了与该类的成员函数加以区别,在声明时前面加以关键字friend。友元不是成员函数,但是它可以访问类中的私有成员。
友元声明只能出现在类定义中。因为友元不是授权类的成员,所以它不受其所在类的声明区域public private 和protected 的影响。
我们可以使用friend关键字在 C++ 中声明一个友元类。
语法:
friend class class_name; // declared in the base class
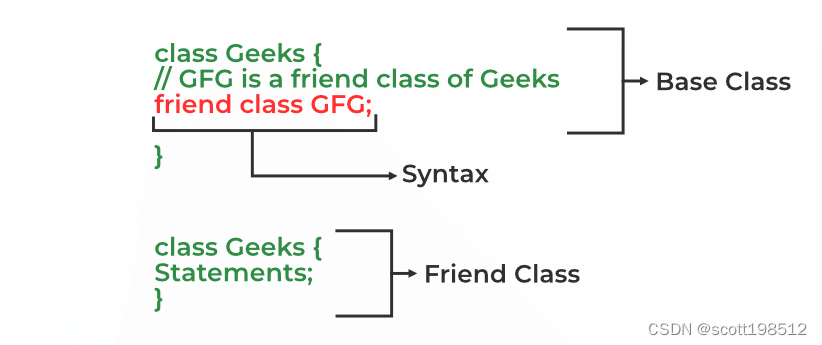
友元类语法
// C++ Program to demonstrate the
// fucntioning of a friend class
#include <iostream>
using namespace std;
class GFG {
private:
int private_variable;
protected:
int protected_variable;
public:
GFG()
{
private_variable = 10;
protected_variable = 99;
}
// friend class declaration
friend class F;
};
// Here, class F is declared as a
// friend inside class GFG. Therefore,
// F is a friend of class GFG. Class F
// can access the private members of
// class GFG.
class F {
public:
void display(GFG& t)
{
cout << "The value of Private Variable = "
<< t.private_variable << endl;
cout << "The value of Protected Variable = "
<< t.protected_variable;
}
};
// Driver code
int main()
{
GFG g;
F fri;
fri.display(g);
return 0;
}
Output:
The value of Private Variable = 10
The value of Protected Variable = 99
注意: 我们可以在基类主体的任何地方声明友元类或函数,无论是私有块、保护块还是公共块。它的工作原理都是一样的。
1.友元功能
与友元类一样,友元函数可以被授予对 C++ 类的私有和受保护成员的特殊访问权限。它们是非成员函数,可以访问和操作类的私有和受保护成员,因为它们被声明为友元。
友元函数可以是:
全局函数
另一个类的成员函数
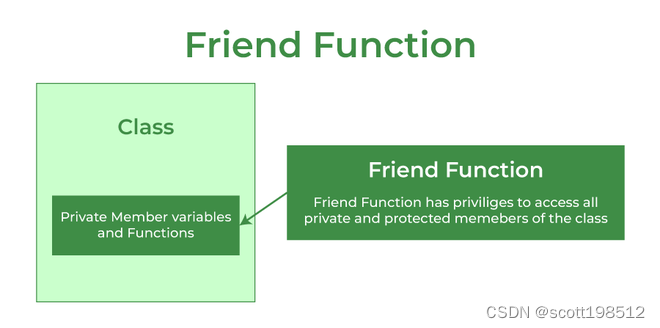
C++ 友元函数
句法:
friend return_type function_name (arguments);// 对于全局函数
//或者
friend return_type class_name::function_name(arguments);// 对于另一个类的成员函数
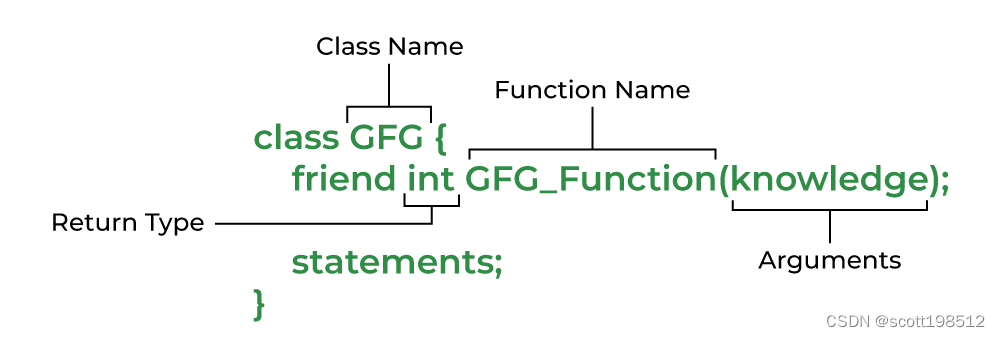
友元函数语法
1.1 全局函数作为友元函数
我们可以将任何全局函数声明为友元函数。以下示例演示如何在 C++ 中将全局函数声明为友元函数:
// C++ program to create a global function as a friend
// function of some class
#include <iostream>
using namespace std;
class base {
private:
int private_variable;
protected:
int protected_variable;
public:
base()
{
private_variable = 10;
protected_variable = 99;
}
// friend function declaration
friend void friendFunction(base& obj);
};
// friend function definition
void friendFunction(base& obj)
{
cout << "Private Variable: " << obj.private_variable
<< endl;
cout << "Protected Variable: " << obj.protected_variable;
}
// driver code
int main()
{
base object1;
friendFunction(object1);
return 0;
}
Output:
Private Variable: << obj.private_variable:10
Protected Variable: << obj.private_variable:99
在上面的例子中,我们使用了一个全局函数作为友元函数。在下一个例子中,我们将使用另一个类的成员函数作为友元函数。
1.2 另一个类的成员函数作为友元函数
我们还可以在 C++ 中将另一个类的成员函数声明为友元函数。下面的例子演示了如何在 C++ 中使用另一个类的成员函数作为友元函数:
// C++ program to create a member function of another class
// as a friend function
#include <iostream>
using namespace std;
class base; // forward declaration needed
// another class in which function is declared
class anotherClass {
public:
void memberFunction(base& obj);
};
// base class for which friend is declared
class base {
private:
int private_variable;
protected:
int protected_variable;
public:
base()
{
private_variable = 10;
protected_variable = 99;
}
// friend function declaration
friend void anotherClass::memberFunction(base&);
};
// friend function definition
void anotherClass::memberFunction(base& obj)
{
cout << "Private Variable: " << obj.private_variable
<< endl;
cout << "Protected Variable: " << obj.protected_variable;
}
// driver code
int main()
{
base object1;
anotherClass object2;
object2.memberFunction(object1);
return 0;
}
Output:
Private Variable: 10
Protected Variable:99
注意 :我们定义另一个类的友元函数的顺序很重要,应该注意。我们总是必须在函数定义之前声明这两个类。这就是为什么我们使用类外定义类成员函数的原因。
1.3友元函数的特点
友元函数是 C++ 中的一个特殊函数,尽管它不是类的成员函数,但它具有访问类的私有和受保护数据的特权。
友元函数是类的非成员函数或普通函数,在类内部使用关键字“ friend ”声明为友元。通过将函数声明为友元,所有访问权限都授予该函数。
关键字“friend”只放在友元函数的函数声明中,不出现在函数定义或调用中。
友元函数像普通函数一样被调用。不能使用对象名称和点运算符调用它。但是,它可以接受该对象作为它想要访问其值的参数。
友元函数可以在类的任何部分声明,即在public或private或protected说明符的模块中声明的效果是一样的。它不受其所在类的声明区域public, private 和protected 的影响。
下面是一些不同场景下友元函数的更多例子:
1.4一个对多个类友好的函数
// C++ Program to demonstrate
// how friend functions work as
// a bridge between the classes
#include <iostream>
using namespace std;
// Forward declaration
class ABC;
class XYZ {
int x;
public:
void set_data(int a)
{
x = a;
}
friend void max(XYZ, ABC);
};
class ABC {
int y;
public:
void set_data(int a)
{
y = a;
}
friend void max(XYZ, ABC);
};
void max(XYZ t1, ABC t2)
{
if (t1.x > t2.y)
cout << t1.x;
else
cout << t2.y;
}
// Driver code
int main()
{
ABC _abc;
XYZ _xyz;
_xyz.set_data(20);
_abc.set_data(35);
// calling friend function
max(_xyz, _abc);
return 0;
}
Output
35
friend 函数为我们提供了一种访问私有数据的方式,但它也有其缺点。下面列出了 C++ 中友元函数的优点和缺点。
2.友元函数的优点
友元函数无需继承类即可访问成员。
friend 函数通过访问它们的private数据充当两个类之间的桥梁。
它可用于增加重载运算符的通用性。
它可以在类的public或private或protected部分中声明。
3.友元函数的缺点
友元函数可以从类外访问类的私有成员,这违反了数据隐藏法则。
友元函数不能在其成员中执行任何运行时多态性。
4.关于友元函数和类的要点
友元应该只用于有限的目的。太多的函数或外部类被声明为具有受保护或私有数据访问的类的友元,这会降低在面向对象编程中封装单独类的价值。
友元不是相互的。如果类 A 是 B 的友元,则 B 不会自动成为 A 的友元。
友元不是世代相传的,也就是不具有持续的继承性。(有关更多详细信息,请参阅此处)
友元的概念在 Java 中是没有的。
相关文章
- C++ 宽字节拷贝
- C++ 定位文件 .text 区段地址
- C++windows内核编程笔记day11 win32静态库和动态库的使用
- qt实现web服务器加载vue应用进行C++和html混合编程-连载【6】-企业级系统开发实战连载系列 -技术栈(vue、element-ui、qt、c++、sqlite)
- C/C++ 去掉字符串首位的空格字符
- C/C++判断大端模式还是小端模式
- 【转】C++ stringstream介绍,使用方法与例子
- 利用C++中的函数getTickCount()和getTickFrequency()测量代码段的运行时间
- 33 C++ - 可重载的运算符
- 【C++入门第二期】引用 和 内联函数 的使用方法及注意事项
- C++中重载与重写函数区别及虚函数(转载)
- C++Primer第五版学习(函数部分 五)特殊用途语言特性
- 基于C++实现(控制台)家谱管理系统【100010501】
- 基于QT(C++)实现(图形界面)通讯录系统【100010321】
- Java vs C++:子类覆盖父类函数时缩小可访问性的不同设计
- C++ 什么是多态
- C++ 虚函数表解析
- C++ and Java template class and function 模板类和模板函数
- VC++适合做什么