如何在pyqt中使用 QStyle 重绘 QSlider
2023-02-18 16:32:02 时间
前言
使用 qss 可以很方便地改变 QSlider
的样式,但是有些情况下 qss 无法满足我们的需求。比如下图所示样式:
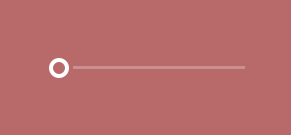
如果直接使用 qss 将 handle 的内圆设置为透明背景,会看到 handle 下面的 groove ,而且画出来的圆环还不圆,如下图所示:

这时候就需要使用 QStyle
来重绘 QSlider
,关于 QStyle
的介绍可以参见 《QStyle设置界面的外观和QCommonStyle继承关系图讲解和使用》,这里不过多赘述(才不是因为我自己也说不清楚)。
实现过程
对于 QSlider
这种比较复杂小部件,需要重写 QProxyStyle
的 drawComplexControl()
和 subControlRect()
,前者决定了 QSlider
的样式,后者用来获取各个子控件所在的矩形区域。为了演示的方便,代码中只重绘了水平滑动条的样式。
- 首先绘制 groove 子控件,从动图中可以看到,要想防止看到 handle 下面的 groove,需将 groove 拆分成两段来绘制:一段是
sub-page
部分,另一段是add-page
部分; - 接着绘制 handle 子控件,handle 子控件有三个部分:透明内圆、不透明圆环以及透明外边距。绘制圆环可以实例化
QPainterPath
,addEllipse()
添加两个不同半径的同心圆之后再painter.drawPath(path)
。为了响应hover
和pressed
,需要分别在opt.activeSubControls == QProxyStyle.SC_SliderHandle
以及widget.isSliderDown()
时更新滑块样式。
下面是具体代码:
# coding:utf-8
from PyQt5.QtCore import QSize, Qt, pyqtSignal, QPoint, QRectF
from PyQt5.QtGui import QColor, QMouseEvent, QPainter, QPainterPath
from PyQt5.QtWidgets import (QProxyStyle, QSlider, QStyle, QStyleOptionSlider,
QWidget)
class HollowHandleStyle(QProxyStyle):
""" 滑块中空样式 """
def __init__(self, config: dict = None):
"""
Parameters
----------
config: dict
样式配置
"""
super().__init__()
self.config = {
"groove.height": 3,
"sub-page.color": QColor(255, 255, 255),
"add-page.color": QColor(255, 255, 255, 64),
"handle.color": QColor(255, 255, 255),
"handle.ring-width": 4,
"handle.hollow-radius": 6,
"handle.margin": 4
}
config = config if config else {}
self.config.update(config)
# 计算 handle 的大小
w = self.config["handle.margin"]+self.config["handle.ring-width"] + \
self.config["handle.hollow-radius"]
self.config["handle.size"] = QSize(2*w, 2*w)
def subControlRect(self, cc: QStyle.ComplexControl, opt: QStyleOptionSlider, sc: QStyle.SubControl, widget: QWidget):
""" 返回子控件所占的矩形区域 """
if cc != self.CC_Slider or opt.orientation != Qt.Horizontal or sc == self.SC_SliderTickmarks:
return super().subControlRect(cc, opt, sc, widget)
rect = opt.rect
if sc == self.SC_SliderGroove:
h = self.config["groove.height"]
grooveRect = QRectF(0, (rect.height()-h)//2, rect.width(), h)
return grooveRect.toRect()
elif sc == self.SC_SliderHandle:
size = self.config["handle.size"]
x = self.sliderPositionFromValue(
opt.minimum, opt.maximum, opt.sliderPosition, rect.width())
# 解决滑块跑出滑动条的情况
x *= (rect.width()-size.width())/rect.width()
sliderRect = QRectF(x, 0, size.width(), size.height())
return sliderRect.toRect()
def drawComplexControl(self, cc: QStyle.ComplexControl, opt: QStyleOptionSlider, painter: QPainter, widget: QWidget):
""" 绘制子控件 """
if cc != self.CC_Slider or opt.orientation != Qt.Horizontal:
return super().drawComplexControl(cc, opt, painter, widget)
grooveRect = self.subControlRect(cc, opt, self.SC_SliderGroove, widget)
handleRect = self.subControlRect(cc, opt, self.SC_SliderHandle, widget)
painter.setRenderHints(QPainter.Antialiasing)
painter.setPen(Qt.NoPen)
# 绘制滑槽
painter.save()
painter.translate(grooveRect.topLeft())
# 绘制划过的部分
w = handleRect.x()-grooveRect.x()
h = self.config['groove.height']
painter.setBrush(self.config["sub-page.color"])
painter.drawRect(0, 0, w, h)
# 绘制未划过的部分
x = w+self.config['handle.size'].width()
painter.setBrush(self.config["add-page.color"])
painter.drawRect(x, 0, grooveRect.width()-w, h)
painter.restore()
# 绘制滑块
ringWidth = self.config["handle.ring-width"]
hollowRadius = self.config["handle.hollow-radius"]
radius = ringWidth + hollowRadius
path = QPainterPath()
path.moveTo(0, 0)
center = handleRect.center() + QPoint(1, 1)
path.addEllipse(center, radius, radius)
path.addEllipse(center, hollowRadius, hollowRadius)
handleColor = self.config["handle.color"] # type:QColor
handleColor.setAlpha(255 if opt.activeSubControls !=
self.SC_SliderHandle else 153)
painter.setBrush(handleColor)
painter.drawPath(path)
# 滑块按下
if widget.isSliderDown():
handleColor.setAlpha(255)
painter.setBrush(handleColor)
painter.drawEllipse(handleRect)
测试
# coding:utf-8
import sys
from PyQt5.QtCore import Qt
from PyQt5.QtGui import QColor
from PyQt5.QtWidgets import QApplication, QWidget, QSlider
class Demo(QWidget):
def __init__(self):
super().__init__()
self.resize(300, 150)
self.setStyleSheet("Demo{background: rgb(184, 106, 106)}")
# 改变默认样式
style = {
"sub-page.color": QColor(70, 23, 180)
}
self.slider = QSlider(Qt.Horizontal, self)
self.slider.setStyle(HollowHandleStyle(style))
# 需要调整高度
self.slider.resize(200, 28)
self.slider.move(50, 61)
if __name__ == '__main__':
app = QApplication(sys.argv)
w = Demo()
w.show()
sys.exit(app.exec_())
相关文章
- [CentOS]查看centos的发行版本情况
- [MySQL]创建用户并指定某一数据库权限
- [PHP] 安装memcached扩展
- [http]301和302的区别
- [MySQL]简单理解并发下的CAS比较交换和ABA问题
- [前端]使用meta控制双核浏览器默认使用webkit/chrome内核
- [PHP] pmap可以查看进程占用内存的详细情况
- [PHP] 解决php中上传大文件的错误
- [日常]GB2312 GBK GB18030的区别和演进过程
- [PHP] 循环查看php-fpm的内存占用情况
- [日常]中文字符串比较大小的方式
- [日常]win10解除网速限制
- [日常]解决win10没有组策略问题
- [MySQL] mysql中bitmap的简单运用
- [MySQL]开启慢查询日志以及未使用索引SQL日志
- [MySQL]explain语句中type字段的具体解释
- [C语言]内存泄漏问题Out Of Memory
- [C语言]内存问题之返回局部变量地址
- [日常]windows 下 CURL SSL CA证书的位置
- [日常]windows系统下使用curl工具