C++ STL:string类的模拟实现
2023-04-18 14:21:37 时间
目录
2.1 获取字符串有效字符数、容量及_str成员变量获取相关函数
前置说明
模拟实现的string类定义了四个成员函数,分别为(1)char* str -- 指向字符串的指针、(2)size_t size -- 字符串中的字符数 、(3)size_t capacity -- 字符串最大可容纳的字符数量、(4)size_t _npos -- size_t类型的-1
一. 构造函数和析构函数的模拟实现
1.1 构造函数
这里实现两种形式的构造函数,一种是通过字符串来构造,第二种是通过一个string类进行拷贝构造。注意:定义拷贝构造函数要进行深拷贝而不是浅拷贝(值拷贝),否则,会使两个string类对象中的_str指向同一块内存区域,在调用析构函数时对同一块空间连续两次释放,从而导致程序崩溃。
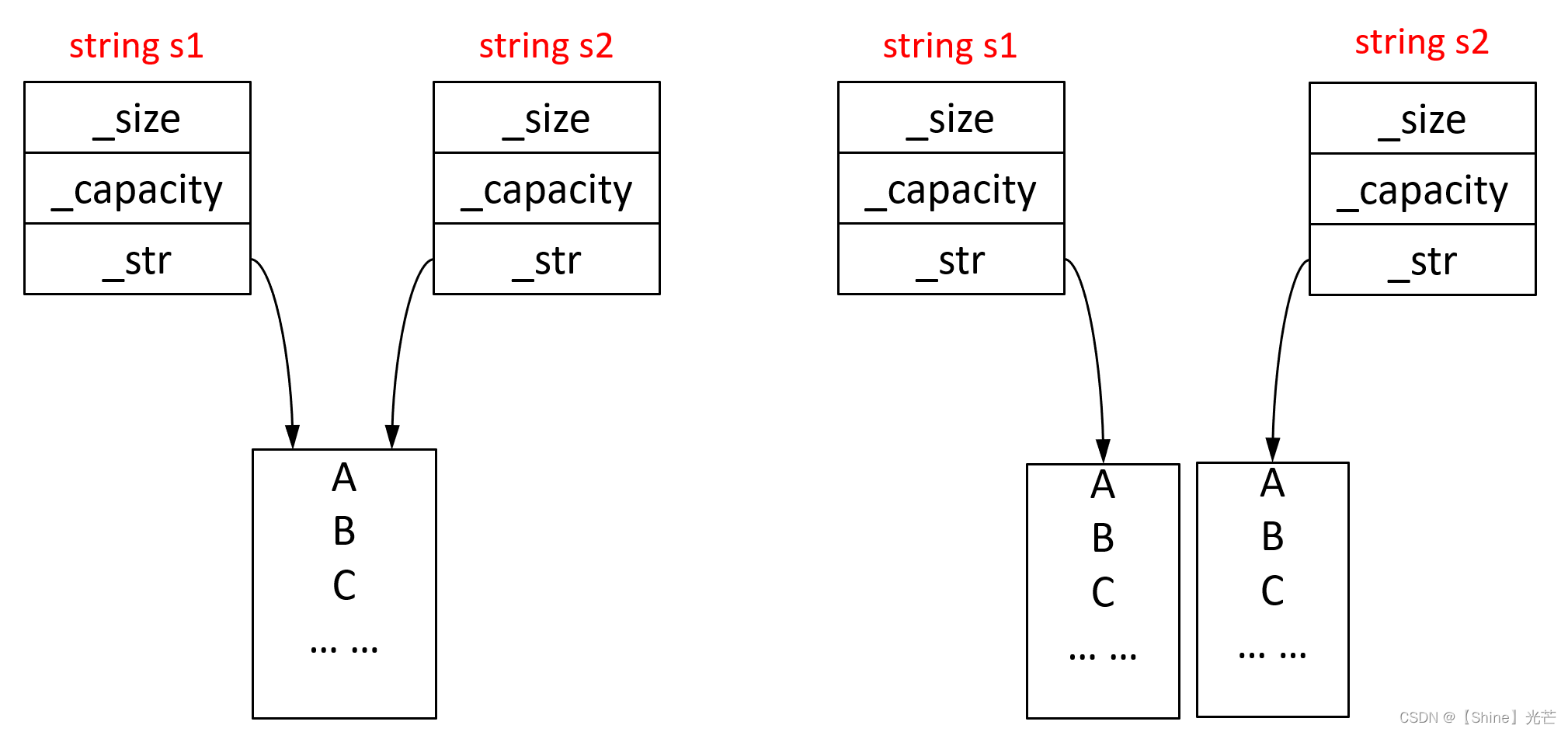
string(const char* str = "") //通过字符串构造和默认构造
: _size(strlen(str))
, _capacity(_size)
{
_str = new char[_capacity + 1];
strcpy(_str, str);
}
//explicit string(const string& str) //拷贝构造
//一般写法 -- 正常完成深拷贝
//string(const string& str) //拷贝构造
// : _size(str._size)
// , _capacity(str._capacity)
//{
// _str = new char[_capacity + 1];
// strcpy(_str, str._str);
//}
//拷贝构造复用写法 -- 临时对象
string(const string& str)
: _str(nullptr)
, _size(str._size)
, _capacity(str._capacity)
{
if (this != &str) //排除自己给自己拷贝的情况
{
string tmp(str._str);
std::swap(_str, tmp._str);
}
}
1.2 析构函数
析构函数要完成的工作为:释放string类对象的成员函数_str指向的内存空间。
~string() //析构函数
{
delete _str;
_str = nullptr;
_size = _capacity = 0;
}
二. string类对象容量及成员相关的函数
2.1 获取字符串有效字符数、容量及_str成员变量获取相关函数
- size函数和length函数:获取字符串长度(末尾'